A. Introduction
if... else allows us to make decisions, this is also called branching in programming. The execution of code is not linear anymore, it branches out and merges back at end of the branches as shown below.
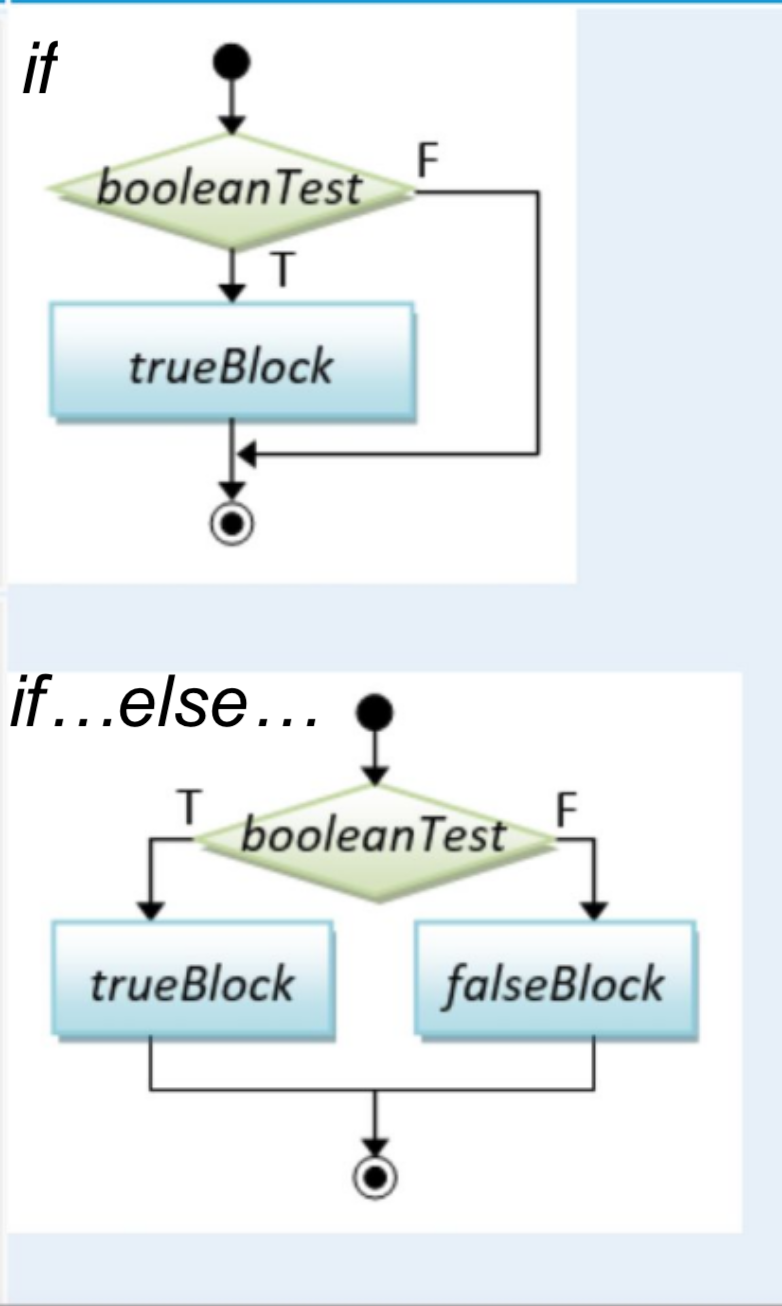
In the "if" flowchart, the "trueBlock" is only executed when "booleanTest" is true. In the "if... else..." flowchart, the "trueBlock" is executed when "booleanTest" is true and "falseBlock" is executed when "booleanTest" is false.
Note here the "trueBlock" and "falseBlock" are referring to the code blocks that are marked by indentations. It's critical to know where each block starts and ends.
B. HW: write if or if... else... statements to solve the problems below. Add comments to indicate where the beginning and end of each "trueBlock" and "falseBlock" are.
Given three integers, print them in ascending order.
Given five integers, print the least of them.
(Optional Challenge) Given the year number. You need to check if this year is a leap year. If it is, print LEAP, otherwise print COMMON.
The rules in the Gregorian calendar are as follows:
a year is a leap year if its number is exactly divisible by 4 and is not exactly divisible by 100
a year is always a leap year if its number is exactly divisible by 400
Note: The words LEAP and COMMON should be printed in all caps.
a = int(input("Please enter integer A: ")) b = int(input("Please enter integer B: ")) c = int(input("Please enter integer C: ")) if (a <= b and a <= c): if (b <= c): print (a, b, c) else: print (a, c, b) elif (b <= a and b <= c): if (a <= c): print (b, a, c) else: print (b, c, a) else: if (a <= b): print (c, a, b) else: print (c, b, a) d = int(input("Please enter integer D: ")) e = int(input("Please enter integer E: ")) f = int(input("Please enter integer F: ")) g = int(input("Please enter integer G: ")) h = int(input("Please enter integer H: ")) if (d <= e and d <= f and d <= g and d <= h): print(d) elif (e <= d and e <= f and e <= g and e <= h): print(e) elif (f <= d and f <= e and f <= g and f <= h): print(f) elif (g <= d and g <= e and g <= f and g <= h): print(g) elif (h <= d and h <= e and h <= f and h <= g): print(h) year = int(input("Please enter year number: ")) if (year % 4 == 0 and year % 100 != 0): print("LEAP") elif (year % 400 == 0): print("LEAP") else: print("COMMON")
x=int(input()) y=int(input()) z=int(input()) if (x<y<z): print (z, y, x) if (x<z<y): print (y, z, x) if (y<x<z): print (z, x, y) if (y<z<x): print (x, z, y) if (z<x<y): print (y, x, z) if (z<y<x): print (x, y, z)