Write a program that takes input of a number in word format like “one” or “four”. Print out the ordinal number for the input (ex: “one” → “first”, “five” → “fifth”)
Write a program that inputs a number and generates an error message if it is not a number. It should keep asking for input until it is valid.
Print this table using lists and for loops:
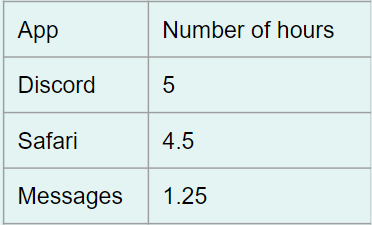
Create a rock-paper-scissors game that uses functions.
Create a .txt file. Write three new lines, then read the entire file.
Complete as you see fit. If you haven't completed the homework before the break yet, please focus on that first!
Resources:
Contact Info:
shravyassathish@gmail.com (mentor)
kingericwu@gmail.com (TA)
felixguo41@gmail.com (TA)
#1
number = input("Please enter a number in word format (one, two, three... five hundred thirty four) oh and please no hyphens ").lower()
number.split()
last = ""
cornerCases = {"one":"first", "two": "second", "three": "third", "five": "fifth", "eight": "eighth", "nine": "ninth", "twelve": "twelfth"}
if number[-1] in cornerCases:
last = cornerCases[number[-1]]
elif number[-1][-1] == "y":
last = number[-1][:-1] + "ieth"
else:
last = number[-1] + "th"
for i in range(0, len(number) - 1):
print(number[i], end = " ")
print(last)
#2
num = input("Please enter a number: ")
while 1:
try:
int(num)
except:
num = input("Please enter a number: ")
else:
break
#3
table = [["App", "Number of hours"], ["Discord", 5], ["Safari", 4.5], ["Messages", 1.25]]
for row in table:
for item in row:
print(item, end = " ")
print("\n")
#4
import random
choices = ["rock", "paper", "scissors"]
def rps():
user = input("Rock, paper, scissors! ").lower()
comp = random.choice(choices)
if comp != user:
if comp == "rock":
if user == "paper":
print("You won!")
else:
print("You lost.")
elif comp == "paper":
if user == "scissors":
print("You won!")
else:
print("You lost.")
else:
if user == "rock":
print("You won!")
else:
print("You lost.")
else:
print("Tie.")
if input("Wanna play rock paper scissors? ").lower() == "yes":
rps()
else:
print("Oh, ok.")
#5
input = open("file.txt", "w")
input.write("Line1\nLine2\nLine3")
input.close()
output = open("file.txt", "r")
print(output.read())
number = input("Part 1: Please input a number to ordinalize (from one to one hundred): ").lower()
irregNumbers = {"one":"first", "two":"second", "three":"third", "five":"fifth", "twelve":"twelfth", "eight":"eighth", "nine":"ninth"}
div10 = {"twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety"}
if number in irregNumbers.keys():
print(f"The associated ordinal number is {irregNumbers.get(number)}")
elif number in div10:
print(f"The associated ordinal number is {number[:-1]}ieth")
else:
try:
if number.split("-")[1] in irregNumbers.keys():
print(f"The associated ordinal number is {number.split('-')[0]}-{irregNumbers.get(number.split('-')[1])}")
except IndexError:
print(f"The associated ordinal number is {number}th")
numbered = False
while numbered == False:
num2 = input("\n\nPart 2: Please input characters to check for numberness: ")
try:
print(f"{float(num2)} is a number!")
numbered = True
except ValueError:
print("That's not a number.")
print("\nPart 3: ")
table = []
def addrow(col1, col2):
table.append([col1, col2])
addrow("App", "Number of hours")
addrow("Discord", "5")
addrow("Safari", "4.5")
addrow("Messages", "1.25")
for list in table:
print(list[0], list[1], sep = "\t")
import random
choices = ("rock", "paper", "scissors")
def rps():
choice = input("\n\nPart 4: Let's play rock, paper, scissors! Rock, paper, scissors, shoot... ").lower()
comp = random.choice(choices)
if choice in choices:
if comp == choice:
print("We drew...")
elif comp == "rock":
if choice == "paper":
print(f"You won! {choice} beats {comp}.")
else:
print(f"You lost. {comp} beats {choice}.")
elif comp == "paper":
if choice == "rock":
print(f"You lost. {comp} beats {choice}.")
else:
print(f"You won! {choice} beats {comp}.")
else:
if choice == "rock":
print(f"You won! {choice} beats {comp}.")
else:
print(f"You lost. {comp} beats {choice}.")
else:
print("Please enter a valid choice.")
again = input("Want to play again? ")
if again.lower() == "yes":
rps()
rps()
with open("temp.txt", "w+") as f:
f.writelines(["three\n", "new\n", "lines!!!\n\n"])
f.seek(0)
print("\n\nPart 5: " + f.read())