A. Introduction
If your expressions calculate square roots, power, absolute values etc, you might need standard functions (also called methods, "functions" and "methods" can be used interchangeably). For instance, if we need to calculate square root, we use a standard method Math.sqrt().
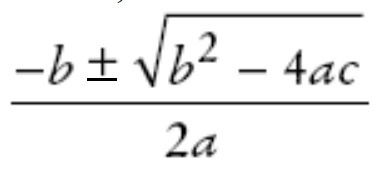
Define three variables, a, b and c. Let a=2, b=-1, c=-21. Please write Java code to solve the values of the express to the left.
B. Standard methods are provided to us in Java Standard Library. We just use them. Common Math Functions(Methods) in Java are listed here:
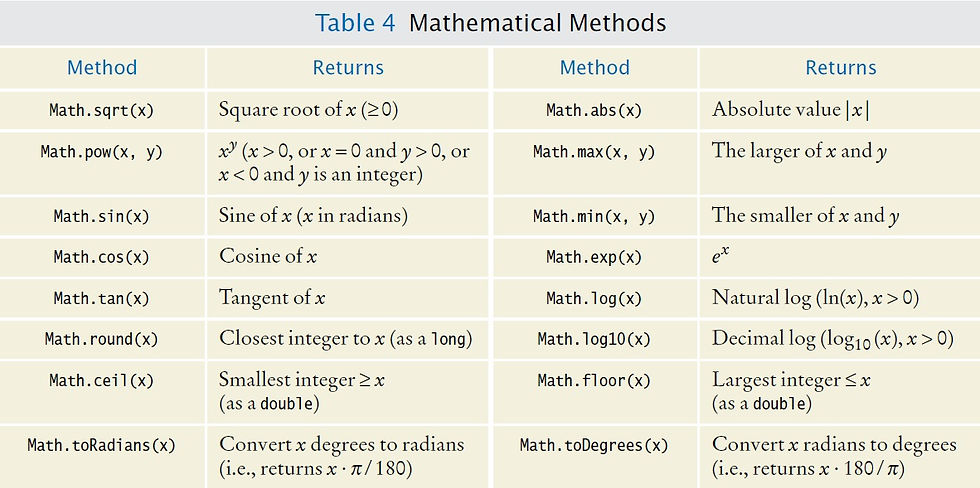
C. Try(HW):
R4.4, R4.5, E4.4
class quadraticEquation {
public static void main() {
int a=2;
int b=-1;
int c=-21;
System.out.println("x=" + ((-b + Math.sqrt(b*b - 4*a*c))/(2*a)) + " or " + ((-b - Math.sqrt(b*b - 4*a*c))/(2*a)));
}
}
x=3.5 or -3.0
double a=2;
double b=-1;
double c=-21;
double d= Math.pow(b, 2)-4*a*c;
double x1=-b+Math.sqrt(d);
double x2=-b-Math.sqrt(d);
double x=x1/(2*a);
double x3=x2/(2*a);
System.out.println(x);
System.out.println(x3);
}
-3.0
3.5
double a=2;
double b=-1;
double c=-21;
System.out.println((-b + Math.sqrt(b*b - 4*a*c))/(2*a)); //Positive solution
System.out.println((-b - Math.sqrt(b*b - 4*a*c))/(2*a)); // Negative solution
//3.5
//-3.0