A. Introduction
We mentioned before each variable or literal has to be of a certain type. But what if you mix different types in an expression? Rule of thumb is all "lower" types will be automatically promoted to the "higher" type in the operations. That's why 1729 / 10 = 172, but 1729 / 10.0 = 172.9. In the first expression, because both 1729 and 10 are int, so the result is int; in the second expression because 10.0 is double, 1729 is "promoted" to double, so the result is double - hence you get 172.9.
This type of conversion happens implicitly - from int to double or float when both types are in the same expression.
B. Explicit Type Casting
Sometimes, if the programmer wants to control the types of a variable or expression, he/she can use "explicit type casting". i.e. specifying the type in front of the variable in a pair of ( ). For instance, (double) 1729 / 10 = 172.0, while ((double) 1729 ) / 10 = 172.9. Similarly, (int)123.45 will valuate to 123. Be mindful with the ( ). See what's wrong below:
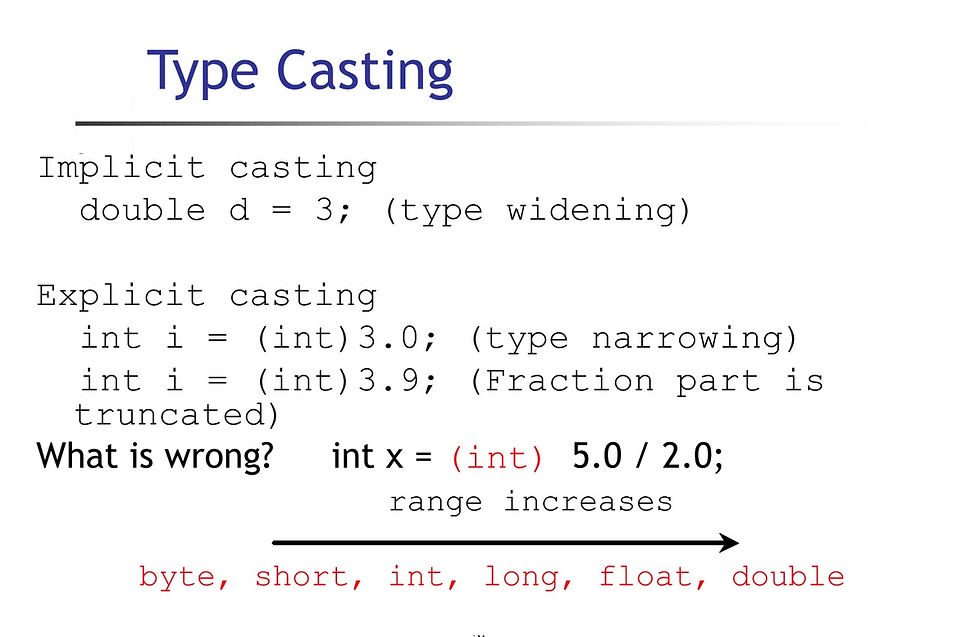
C. Try
R4.9 What are the values of the following expressions, assuming that n is 17 and m is 18?
a. n / 10 + n % 10
b. n % 2 + m % 2
c. (m + n) / 2
d. (m + n) / 2.0
e. (int) (0.5 * (m + n))
f. (int) Math.round(0.5 * (m + n))