A. Introduction
We said in 1.5 that when writing a program, you start from an algorithm, which is the series of steps to solve your problem, and can be written in pseudo code. For instance, to solve the following problem:
You put $10,000 into a bank account that earns 5 percent interest per year. How many years does it take for the account balance to be double the original?
you can use this algorithm (written in pseudo code, of course there are other ways):
Start: year = 0, rate=0.05, balance = 10,000
Repeat steps below until balance reaches 20,000
Add 1 to the year value;
Compute the interest as balance x 0.05 (i.e., 5 percent interest);
Add the interest to the balance
Print the final value of year
B. Try:
1. Suppose your cell phone carrier charges you $29.95 for up to 300 minutes of calls, and $0.45 for each additional minute, plus 12.5 percent taxes and fees. Give an algorithm to compute the monthly charge from a given number of minutes. What should replace "?" in the following pseudo code?
Start: set number of minutes to x;
If x ? 300 then charge = 29.95
else charge = 29.95+(x-300)*0.45
set tax = ? * charge (i.e., 12.5% )
set total charge = ?
Print out total charge
2. Consider the following pseudo code for finding the most attractive photo.
Line up all photos in a row;
Pick the first photo and call it "the best so far";
For each photo in the row, repeat the following till reach the end of row:
If it is more attractive than the "best so far":
discard "the best so far"
call this photo "the best so far"
else do nothing (well, it's okay to omit this line)
Move to the next photo
The photo called "the best so far" is the most attractive photo
Is this an algorithm that will find the most attractive photo? Can you modify this algorithm to find the most ugly photo? Donald Trump's photo? the biggest number? the smallest number?
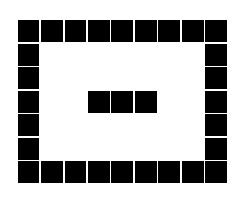
3. Write an algorithm to create a tile pattern composed of black and white tiles, with a fringe of black tiles all around and two or three black tiles in the center, equally spaced from the boundary.
The inputs to your algorithm are the total number of rows x and columns y. (x=7, y=9 is shown here. You can try x=6, y=8 or x=4, y=5, see how the pattern will change)
4. Given an integer, print out each digit of that integer.
5. Given a 16-digit credit card number, print out the sum of all digits.
class Algorithm {
public static void main(String[] args) {
int x = 12;
int y = 8;
System.out.println("********")
for ( int i=0; i< x-2 ; i++)
System.out.println("* *");
System.out.println("********");
}
}
3. Write an algorithm to create a tile pattern composed of black and white tiles, with a fringe of black tiles all around and two or three black tiles in the center, equally spaced from the boundary.
Amount of rows = x
Amount of columns = y
Amount of spacing between side tiles and center tiles = z
Repeat the following step for y times
Print out one black tile
Move to the next row
Repeat the following steps for (x-3)/2 times
Print out one black tile
Repeat the following steps for y-2 times
Print out one white tile
Print out one black tile
Move to next row
Repeat the following for x-2-(2*((x-3)/2)) times
Print out one black tile
Repeat the following step for z times
Print out one white tile
Repeat the following step for x-2-2*z times
Print out one black tile
Repeat the following step for z times
Print out one white tile
Print out one black tile
Move to next row
Repeat the following steps for (x-3)/2 times
Print out one black tile
Repeat the following steps for y-2 times
Print out one white tile
Print out one black tile
Move to next row
Repeat the following step for y times
Print out one black tile
4. Given an integer, print out each digit of that integer.
user input = integer
Amount of digits in the integer = #digits
Repeat the following steps for #digits times
Print out the last digit of the most current form of integer
cut the last digit (just counted) off from the most current form of integer
5. Given a 16-digit credit card number, print out the sum of all digits.
sum of all digits = sum
16-digit credit card number = integer
Repeat the following steps for 16 times
add the last digit of the most current form of integer to sum
cut the last digit (just counted) off from the most current form of integer
Print out sum
let “r” be the # of black tiles in each row; r = 7
let “c” be the # of black tiles in each column; c = 9
let “a1” be the midpoint of the vertical lines
let “a2” be the midpoint of the horizontal lines
make a single row of 9 black tiles
on 9th tile, place down (r-1) black tiles vertically downwards
on lowest vertical tile, make a row of (c-1) black tiles
on leftmost tile of the lowest horizontal line, place down (r-2) black tiles upwards
find a1 and a2
for leftmost vertical line, move the midpoint a2 / 2 units to the right
for rightmost vertical line, move the midpoint a2 / 2 units to the left
for the uppermost horizontal line, move the midpoint a1 / 2 units down
for the bottomost horizontal line, move the midpoint a1 / 2 units up
find the point where all 4 midpoints overlap into a single midpoint
1.5 Robot:
Set int r = number of rows / Set int c = number of columns/ For the the first row, print c blocks. Then "\n" or skip a line. Determine x, y, m, and n./ If c is even, then int m = 2/ If c is odd, then int m = 3/ If r is even, then int n = 2/ If r is odd, then int n = 1/ If c is even, then x = c/2 - 2/ If c is odd, then x = c/2 - 1.5/ If r is even, then y = r/2 -2./ If r is odd, then y = r/2-1.5/ Print one block, (c-2) blank spaces, another block, and skip a line. Repeat y times. Then, print one block, x spaces, m blocks, x spaces, another block, and skip a line. Repeat n times. Subsequently, print one block, (c-2) blank spaces, another block, skip a line, and repeat that y times. Finally, print c blocks. Then System.out.println("THIS IS BETTER THAN WHAT HENRY DID!")
columns: x=7
row's: y=9
Make a row that has 9 black squares all next to each other
then on the 9th black square make a column with 6 more black squares next to each other
then on the last square you made on your column make 8 more black squares next to each other in a row
then on the last square you made on your second row make a column with 5 more black squares next to each other
then on the point x=4 and y=4 make a black square
then make two more black squares to the right of that
Rows: x is 6
columns: y is 8
First, make a row that has y black tiles at the top. Once you reach the end of the row, place (x-1) black tiles as you go down. Then, put down (y-1) black tiles as you go to the left. Next, place (x-2) black tiles as you go up. For the last few step, start by going (y-4) to the right and (x-2) down. Place a black tile on your current location and the tile to the right.
2nd algorithm
Number of rows = x is 6
Number of columns = y is 8
Place y black tiles rightward on the first row;
Place (x - 1) black tiles going down;
At the last tile drawn, place (y - 1) black tiles to the left;
At the last tile drawn, place (y - 2) black tiles upwards then go one black tile up;
Go (x - 4) down then (y - 3) to the right;
Then draw 2 black tiles to the right;
Go to the left 4 black tiles;
Then go down 1 tile down;
Then go (y - 3) to the right;
Then draw 2 black tiles to the right;
1st Algorithm
Number of rows = x is 7
Number of columns = y is 9
Place y black tiles rightward on the first row;
Place (x - 1) black tiles going down;
At the last tile drawn, place (y - 1) black tiles to the left;
At the last tile drawn, place (y - 2) black tiles upwards then go one black tile up;
Go half of (x-1) down and (y-2-3)/2 right
Then draw 3 black tiles to the right;
If first row or last row, fill all spaces with color
Fill in the first and last columns with color
Take the total number of rows and subtract 2 and divide that number by 2, if that number is a fraction round down.
Add that number to the 1st row and subtract that number form the last row. The remaining range will be the middle repeat this process for the columns and fill in the middle according to the ranges.
Number of rows = #rows
Number of Columns = #clms
Place #clms black tiles
Go down 1 line
Place 1 black tile
Place #clms - 2 white tiles
Place 1 black tile
Go down 1 line
Repeat the previous 4 steps x - 1 times
If #rows is even:
x = (#rows / 2) - 2
Else:
x = (#rows / 2) - 1.5
Place 1 black tile
Place x white tiles
Place #clms - (2 + 2x) black tiles
Place x white tiles
Place 1 black tile
Go down 1 line
If #rows is even:
repeat the previous 6 steps
Place 1 black tile
Place #clms - 2 white tiles
Place 1 black tile
Go down 1 line
Repeat the previous 4 steps x - 1 times
Place #clms black tiles