A. Introduction
Have you completed all the tasks in 1.3? If not, try to do all of them. Make sure you are comfortable with errors. Now think about it: what else can you program other than basic prints and calculations?
B. Algorithms and Pseudo Code
When dealing with complex problems, you have to think of the steps to solve the problem. When you write down these steps, you create an "algorithm" to solve the problem.
You don't write your algorithms in a programming language, you write them in English using a simplified format such as if... then... else so everyone can understand. The algorithms written in simplified English are called pseudocode. See examples here.
C. Try this:
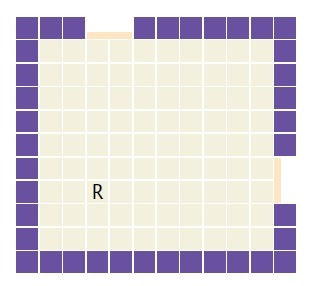
Consider a robot that is placed in a room. The robot can:
• Move forward by one unit. • Turn left or right. • Sense what is in front of it: a wall, a window, or neither.
Write an algorithm that enables the robot, placed anywhere in the room, to count the number of windows. For example, in the room at right, the robot (marked as R) should find that it has two windows.
let “w” be the # of windows. set w = 0
make robot go to wall
turn until robot can sense wall
move the robot around the perimeter of the room with the sensor still facing the perimeter
if sense wall, move to next unit
if sense window, do (w+1), then continue to move around the perimeter
repeat step 4,5 for every unit until you finish the entire perimeter of the room