1. Finish this problem we started in class:
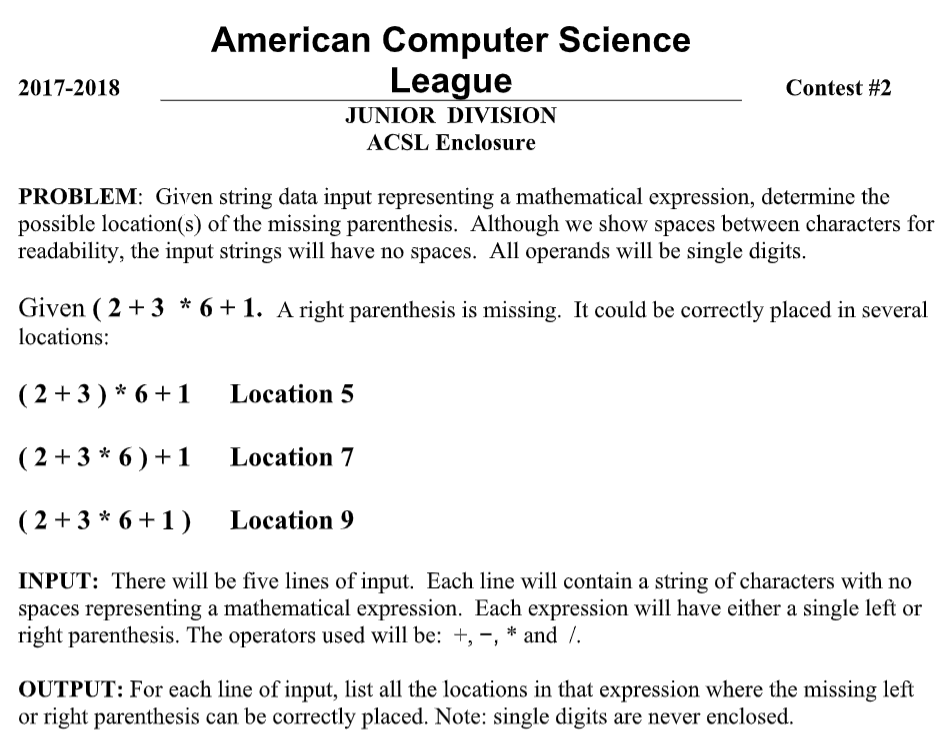
Sample input:
(2+3*6+1
2-5*(6+1
5+5–2)*5
3*5+(8/4*2
2+8/4*5)
Sample output:
7, 9
9
1, 3
9, 11
1, 3, 5
Test input:
6 + 2 / 3 * 4 )
( 2 – 2 + 2 + 3 * 4 / 2
8 / ( 2 + 3 – 6 + 2
7 – 5 + 8 * 6 / 2 ) + 1
9 + 6 ) / 2 – 4 + 5
Test output:
1, 3, 5
5, 7, 9, 11, 13 7, 9, 11
1, 3, 5, 7
1
2. Evaluate the next item popped in this sequence if it were a queue, and then if it were a stack:
PUSH(C) POP(X) PUSH(U) PUSH(Y) POP(X) PUSH(X) PUSH(H) PUSH(G) PUSH(A) PUSH(Q) POP(X) POP(X) PUSH(N) PUSH(L) POP(X) PUSH(E) PUSH(F) POP(X) POP(X)
final_answer = '' for i in range(0, 5): locations = [] expression = input() if '(' in expression: parenthesis = '(' elif ')' in expression: parenthesis = ')' paren_location = int(expression.index(parenthesis)) i = 0 for char in expression: if char.isdigit(): index = int(expression.index(char, i)) if parenthesis == '(': if index > paren_location and abs(index - paren_location) >= 2: locations.append(str(int(index + 2))) elif parenthesis == ')': if index < paren_location and abs((index - paren_location)) >= 2: locations.append(str(int(index + 1))) i += 1 for num in locations: if locations.index(num) == len(locations) - 1: final_answer += num + '\n' else: final_answer += num + ', ' print(final_answer)
2) Queue: Q
Stack: N
https://repl.it/@offexe/EminentUnripeNetframework
As a queue: Q As a stack: N
- William Li
2.queue:Q stack:N
https://www.codiva.io/p/fa67b394-dd7b-4df0-af39-445de80731a8