Finish the coding problem we started in class
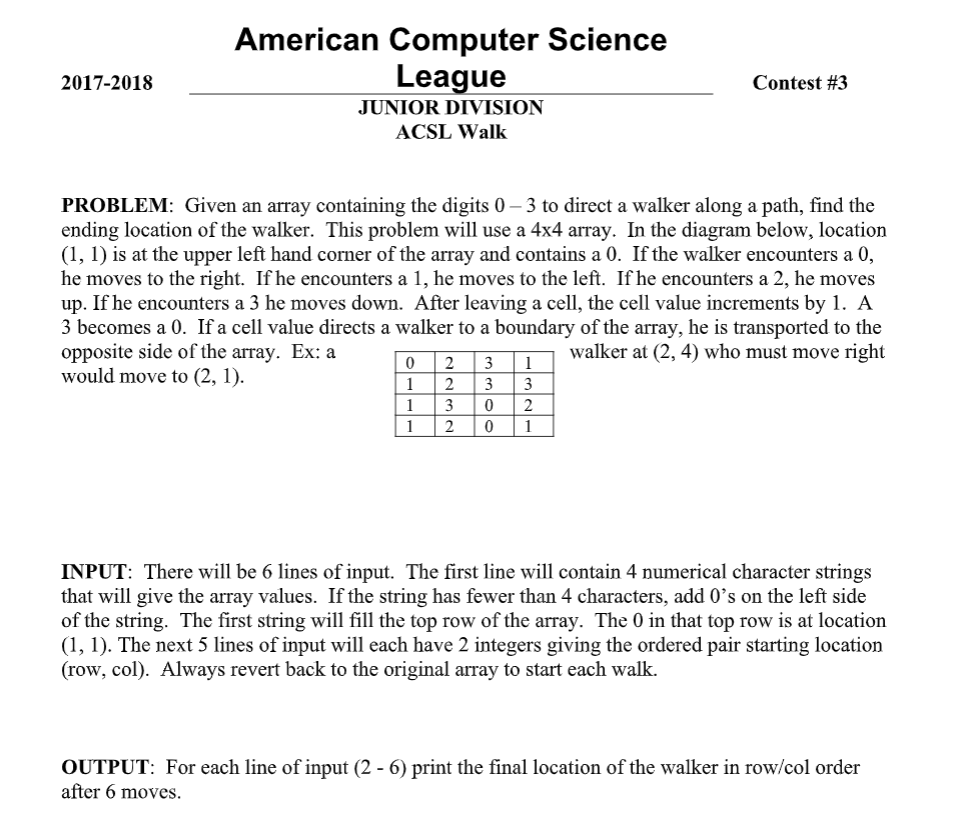
Here is the code we started in Python:
Taking input:
board = []
line = input().split(", ")
i = 0
for i in range(0,4):
board.append([])
while len(line[i])<4:
line[i] = "0"+line[i]
for j in line[i]:
board[i].append(int(j))
Moving the walker once:
copy = [row[:] for row in board] #this creates a copy of the list board
coords = input().split(", ")
r = int(coords[0])-1
c = int(coords[1])-1
if copy[r][c]==0:
copy[r][c] = (copy[r][c]+1)%4
c = (c+1)%4
elif copy[r][c]==1:
copy[r][c] = (copy[r][c]+1)%4
if c==0:
c = 3
else:
c-=1
elif copy[r][c]==2:
copy[r][c] = (copy[r][c]+1)%4
if r==0:
r = 3
else:
r-=1
elif copy[r][c]==3:
copy[r][c] = (copy[r][c]+1)%4
r = (r+1)%4
Here is the code we started in Java:
Taking input:
Scanner in = new Scanner(System.in);
int[][] board = new int[4][4];
String[] line = in.nextLine().split(", ");
for(int i=0; i<4; i++){
while(line[i].length()<4){
line[i] = "0" + line[i];
}
for(int j=0; j<4; j++){
board[i][j] = line[i].charAt(j)-'0';
}
}
Moving the walker once:
int[][] copy = new int[4][];
for(int j=0; j<4; j++){
copy[j] = board[j].clone(); #this creates a copy of the array board
}
String[] coords = in.nextLine().split(", ");
int r = Integer.parseInt(coords[0])-1;
int c = Integer.parseInt(coords[1])-1;
for(int j=0; j<6; j++){
if(copy[r][c]==0){
copy[r][c] = (copy[r][c]+1)%4;
c = (c+1)%4;
}
else if(copy[r][c]==1){
copy[r][c] = (copy[r][c]+1)%4;
if(c==0) c = 3;
else c--;
}
else if(copy[r][c]==2){
copy[r][c] = (copy[r][c]+1)%4;
if(r==0) r = 3;
else r--;
}
else if(copy[r][c]==3){
copy[r][c] = (copy[r][c]+1)%4;
r = (r+1)%4;
}
Code is attached below:
https://www.codiva.io/p/1b100090-de2d-4a46-bfdb-8837ccc2463f
board = [] array = input().split(', ') for item in array: while len(item) < 4: item = "0" + item line = [] for char in item: line.append(int(char)) board.append(line) for i in range(0, 5): copy = [row[:] for row in board] coords = input().split(', ') r = int(coords[0]) - 1 c = int(coords[1]) - 1 for j in range(0, 6): if copy[r][c] == 0: copy[r][c] = (copy[r][c] + 1) % 4 if c == 3: c = 0 else: c += 1 elif copy[r][c] == 1: copy[r][c] = (copy[r][c] + 1) % 4 if c == 0: c = 3 else: c -= 1 elif copy[r][c] == 2: copy[r][c] = (copy[r][c] + 1) % 4 if r == 0: r = 3 else: r -= 1 elif copy[r][c] == 3: copy[r][c] = (copy[r][c] + 1) % 4 if r == 3: r = 0 else: r += 1 print(str(r + 1) + ', ' + str(c + 1))