A. What are reserved words? What are identifiers? What are some of the basic conventions of identifiers?
Answer:
1. Words such as class, public, static, void, and main have specific meanings in Java, they called reserved words or keywords.
2. An identifier is a name for a variable, parameter, constant, user-defined method etc. In Java an identifier is any sequence of letters, digits, and the underscore
3. Identifiers may not begin with a digit; Identifiers are case-sensitive; Wherever possible identifiers should be concise and self-documenting. A variable called "area" is more illuminating than one called "a".
4. By convention identifiers for variables and methods are lowercase. Uppercase letters are used to separate these into multiple words, for example getName, findSurfaceArea, preTaxTotal, and so on. Note that a class name starts with a capital letter. Reserved words are entirely lowercase and may not be used as identifiers.
B. What are primitive types? How are integers stored in computer?
Answer:
1. Java has 8 primitive types: byte, short, int, long, float, double, boolean and char
2. Integer values in Java are stored exactly, as a string of bits (binary digits 0 or 1). One of the bits stores the sign of the integer, 0 for positive, 1 for negative.
3. The primitive type byte uses one byte (eight bits) of storage; int uses 4 bytes, short uses 2 bytes and long uses 8 bytes.
C. How are floating-point number stored? What is mantissa? What are compounded round-off errors?
Answer:
1. A floating-point number is stored in two parts using scientific notation: a mantissa, which specifies the digits of the number, and an exponent.
2. In type double, there are 64 bits in total. 11 bits are allocated for the exponent, and (typically) 52 bits for the mantissa. 1 bit is allocated for the sign. This is a double-precision number.
3. When floating-point numbers are converted to binary, most cannot be represented exactly, leading to round-off error. These errors are compounded by arithmetic operations.
D. What is the best way to compare two double floating-point numbers?
Answer:
Because of round-off errors, when comparing floating-point numbers such as x and y, you should check the relative difference instead of x==y or x!=y. Traditionally, if |x-y| is "small enough" (typically |x-y| <= 10^ -16 * max(|x|, |y|)), it is considered x==y.
E. What is the difference among NaN, Infinity and -Infinity?
Answer:
1. NaN, “not a number.” means undefined result. Examples of operations that produce NaN are: taking the square root of a negative number, or 0.0 divided by 0.0.
2. An operation that gives an infinitely large or infinitely small number, like division by zero, produces a result of Infinity or -Infinity
F. What are final variables?
Answer
1. A final variable identified by the keyword final, is used to name a quantity whose value will not change once assigned.
2. Here are some examples of final declarations:
final double TAX_RATE = 0.08;
final int CLASS_SIZE = 35;
...
final double TAX_RATE;
if (< some condition >)
TAX_RATE = 0.08;
else
TAX_RATE = 0.0;
// TAX_RATE can be assigned a value just once: its value is final!
G. What are prefix for binary, hexadecimal and octal literals? How to convert them ?
Answer:
1. 0b or 0B is the prefix to indicate binary literals; 0x or 0X is the prefix to indicate hexadecimal literals; 0 is the prefix for octal literals. (Literals are constants)
2. To convert between base 2 and base 10, see here
3. To convert among base 2, base 8 and base 16, see here
H. Practice of the Week:
1.
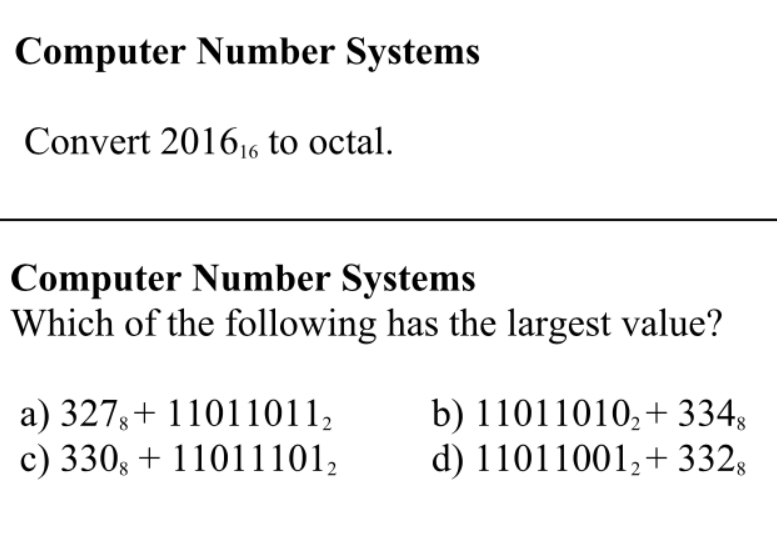
2.
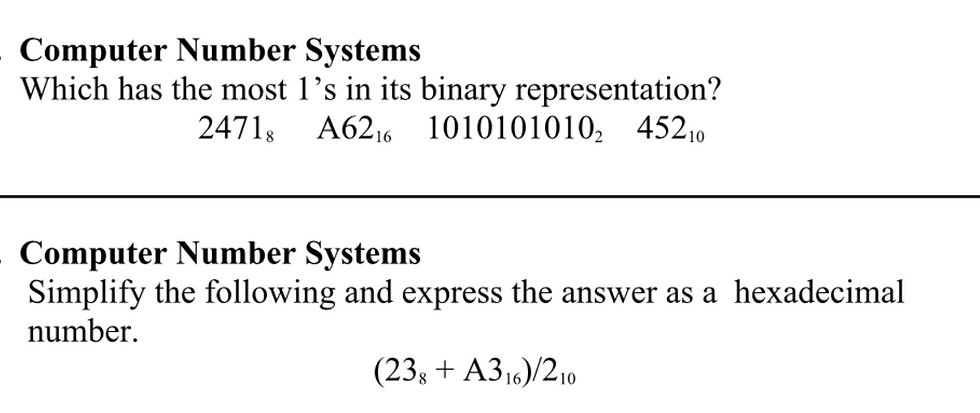
3. Q18-20 of Chapter 2 in Barron's (edition 8)
20026
1/15/2020 tyler
1. a) 2016 (hexa) --> 0010 0000 0001 0110 (binary)--> 000 010 000 000 010 110 (binary)--> 020026 (octal)--> 20026 octal
b) a. 327 (octal) + 011 011 011 (bi) --> 327 + 333 --> 777(octal)
b. 011 011 010 (bi) + 334 (octal) --> 332 + 334 --> 666(octal)
c. 330 (octal) + 011 011 101 (bi) --> 330+335 --> 665(octal)
d. 011 011 001 (bi) + 332 (octal) --> 331 + 332--> 663(octal)
Most 1's: 2471 (octal)
2471 (octal) --> 010 100 111 001 --> 6 ones
A62 (hexa) --> 1010 0110 0010 --> 5 ones
1010101010 (bi) --> 5 ones
454 (deca) -->
454/2 = 226 0
226/2 = 118 0
118/2 = 59 0
59/2 = 29 1
29/2 = 14 1
14/2 = 7 0
7/2 = 3 1
3/2 = 1 1
1/2 = 0 1
--> 111011000 --> 5 ones (454 deca)
Simplify: 5B
( (2*8^1 + 3*8^0) + (10*16^1 + 3*16^0) )/2 = (3+16 + 160+3) /2 = 182/2 = 91 (deca)
91 (deca) -->
91/16=5 B
5/16=0 5
--> 5B (hexa)
2. 18) B
19) C
20) D
21)