FRQ 2. Consider the hierarchy of classes shown in the following diagram.
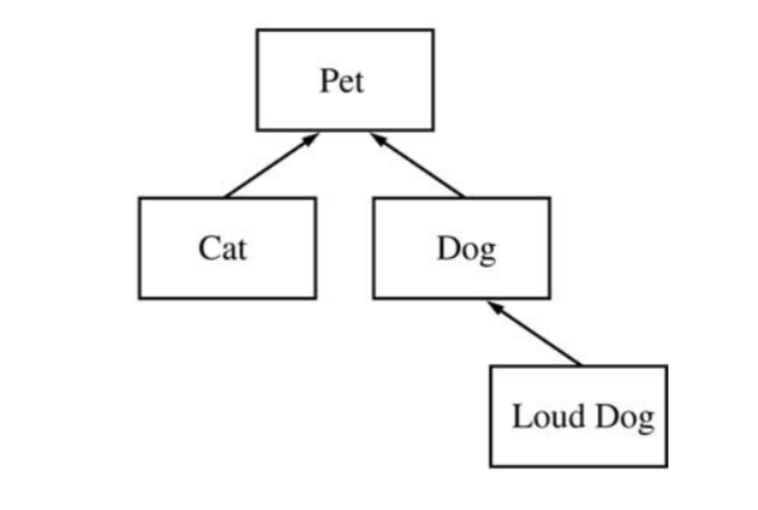
Note that a Cat “is-a” Pet, a Dog “is-a” Pet, and a LoudDog “is-a” Dog.
The class Pet is specified as shown in the following declaration. Each Pet has a name that is specified when it is constructed.
public class Pet
{
private String myName;
public Pet(String name) { myName = name; }
public String getName() { return myName; }
public String speak() {return ""; }
}
The subclass Dog has the partial class declaration shown below.
public class Dog extends Pet
{
public Dog(String name) { /* implementation not shown */ }
public String speak() { /* implementation not shown */ }
}
(a) Given the class hierarchy shown above, write a complete class declaration for the class Cat, including implementations of its constructor and method(s). The Cat method speak returns "meow" when it is invoked.
(b) Assume that class Dog has been declared as shown at the beginning of the question. If the String dog-sound is returned by the Dog method speak, then the LoudDog method speak returns a String containing dog-sound repeated two times.
Given the class hierarchy shown previously, write a complete class declaration for the class LoudDog, including implementations of its constructor and method(s).
(c) Consider the following partial declaration of class Kennel.
public class Kennel
{
private ArrayList petList; // all elements are references
// to Pet objects
// postcondition: for each Pet in the kennel, its name followed
// by the result of a call to its speak method
// has been printed, one line per Pet
public void allSpeak()
{ /* to be implemented in this part */ }
// ... constructor and other methods not shown
}
Write the Kennel method allSpeak. For each Pet in the kennel, allSpeak prints a line with
the name of the Pet followed by the result of a call to its speak method.
In writing allSpeak, you may use any of the methods defined for any of the classes specified for this problem. Assume that these methods work as specified, regardless of what you wrote in parts (a) and (b).
Solutions that reimplement functionality provided by these methods, rather than invoking these methods, will not receive full credit. Complete method allSpeak below.
// postcondition: for each Pet in the kennel, its name followed
// by the result of a call to its speak method
// has been printed, one line per Pet
public void allSpeak()
https://replit.com/@offexe/Animals/