A. Introduction
There is actually an ultimate superclass to all the classes a programmer creates, which is the Object class. Essentially, every class automatically extends Object, which means that Object is a direct or indirect superclass of every other class.
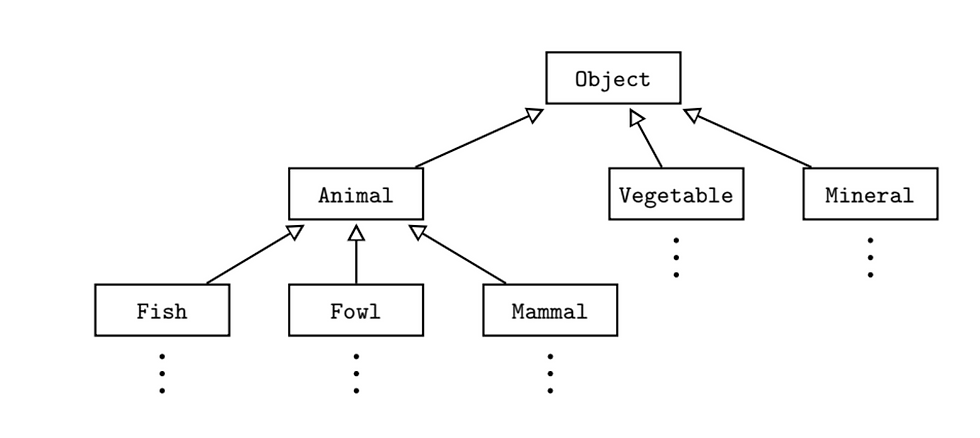
Why so?
B. Methods in Object class
The object class provides some useful methods that are common to all objects. The expectation is that these methods will be overridden in any class where the default implementation is not suitable. For example,
1. The toString method: public String toString()
When you attempt to print an object, the inherited default toString method is invoked, and what you will see is the class name followed by an @ followed by the address in memory of the object, which is typically a meaningless number. This method is meant to be overridden to provide a meaningful way to return a String form of the object. System.out.println() method always call toString() to print out an object, even if your final program doesn’t need to output any objects, overriding toString() is always a good practice for debugging.
2. The equals method: public boolean equals(Object other)
Recall in the following snippet, temp1 is only an alias, the assignment does NOT create a new object. So when comparing if two objects are equal, == only compares the object references, not the object contents.
class Main {
public static void main(String[] args) {
Employee[] team = new Employee[3];
team[0] = new HourlyEmployee("Anthony", 13);
team[1]= new SalariedEmployee("Ryan", 230000);
team[2] = new Manager("Ian Chen", 200000, 1000);
Employee temp1 = team[2];
Employee temp2 = new Manager("Ian Chen", 200000, 1000);
for(Employee x: team)
{
System.out.print(x.weeklyPay());
System.out.println(" is this week's pay for "+x.getName());
}
System.out.println(230000/52.0+90);
}
}
To test if the contents of temp2 and team[2] are equal, you have to override the equals() method in the Employee class to achieve this.
C. Try this: Question 7-10 on page 190 of Barron's AP CS A
7. E
8. D
9. A
10. C