A. Introduction
We have discussed the variables and constructors of the Employee class hierarchy, now it's time to think about the methods.
Other than setters and getters, the key method is weeklyPay(). How do we allow HourlyEmployee, SalariedEmployee, and Manager class to calculate weekly wage using different formulas in the same method? This is a typical case for method overriding.
B. Watch(Slides)
Parent-child relationship rules #4: to override a parent's public method, the method with the exact same signature must be re-defined in a subclass.
C. Try this:
1. Refer to the class hierarchy below. Design class Animal, Dog, Cat and Cow. Implement a method "public String say( )" in each class to allow a dog to say "Woof", a cat to say "Meow" and a cow to say "Moo". Create a Tester class to instantiate an object of each subclass and test the say() method.
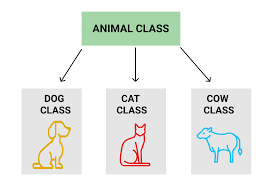
2. In the current Question/ChoiceQuestion/MultipleChoiceQuestion classes, what will happen if you remove "super." in line 13?
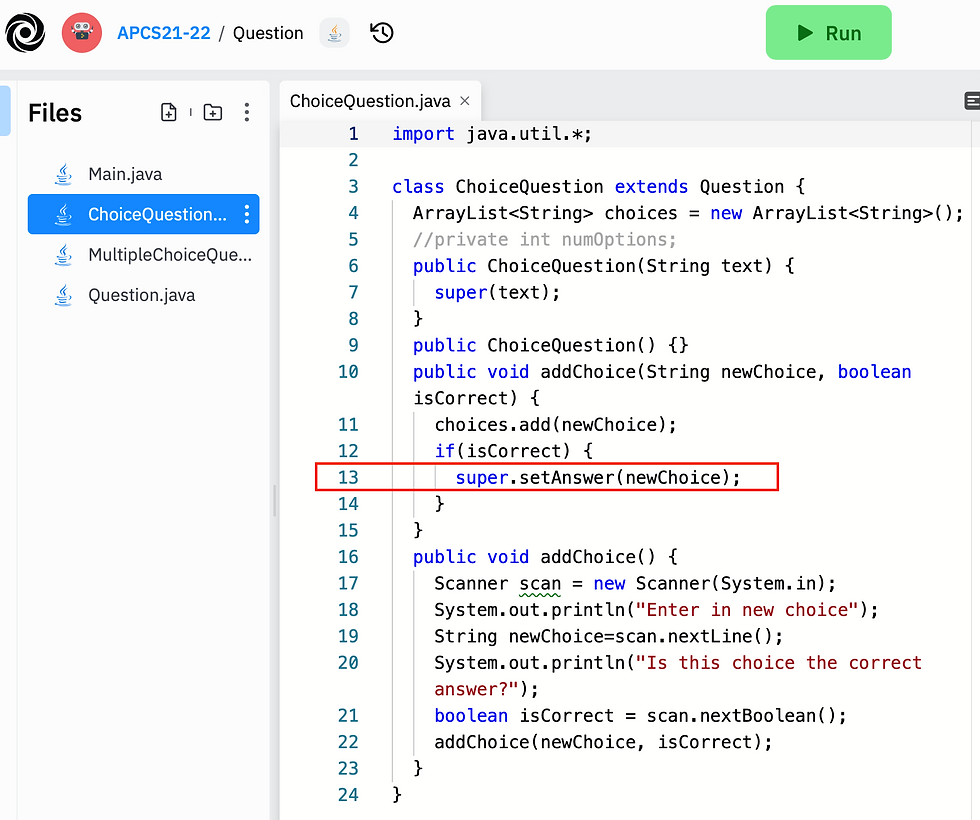
3. Suppose q is an object of the class Question and cq an object of the class Choice-
Question. Which of the following calls are legal?
a. q.setAnswer(response)
b. cq.setAnswer(response)
c. q.addChoice(choice, true)
d. cq.addChoice(choice, true)
4. In class ChoiceQuestion.java, override public void display() to display all choices of a question right after the question text.
5. Add a method addText to the Question superclass and provide a different implementation of ChoiceQuestion that calls addText rather than storing an array list of choices.
6. Refer to the class hierarchy below. Design class Shape, TwoDimentionalShape, Circle, Square and Triangle. Implement two methods "public double perimeter( )" and "public double area()" in all five classes to return correct perimeters and areas for all two dimentional shapes.

https://replit.com/@offexe/6-5#Questions.md