A. Notes about "this" and "super"
super and super() are different. While super() is the call to the superclass constructor, super is the reference to the superclass object.
If presented, super() has to be the first line in the subclass constructor. If not presented, it is implied to be the first line of the subclass constructor.
Similar to this.name, "super" can be used to point to the super class object, such as super.text or super.setText().
B. Here is another hierarchy to practice the relationship between super and sub classes.
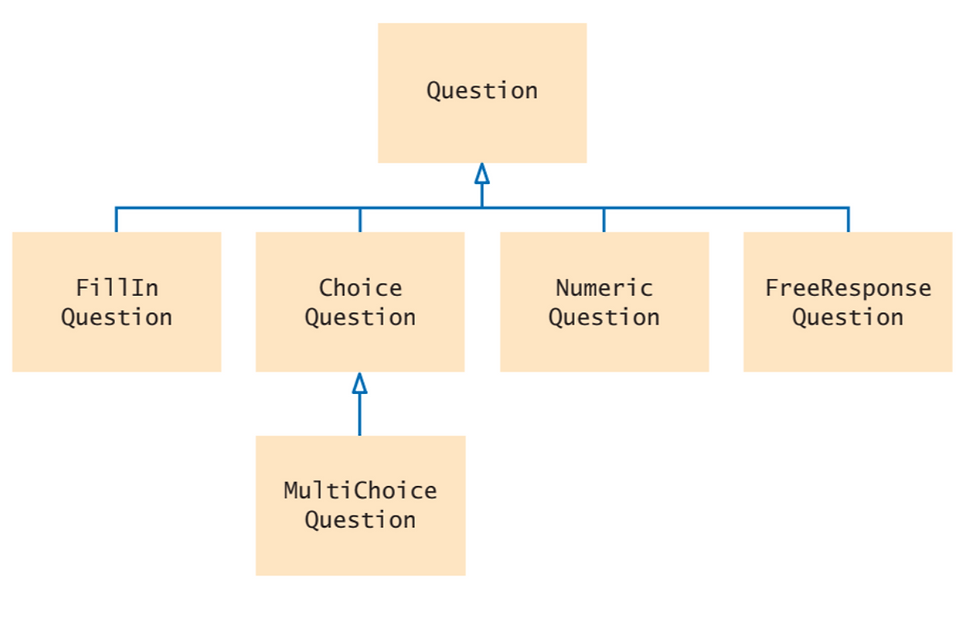
The parent class Question could look like this:
1 /**
2 A question with a text and an answer.
3 */
4 public class Question
5 {
6 private String text;
7 private String answer;
8
9 /**
10 Constructs a question with empty question and answer.
11 */
12 public Question()
13 {
14 text = "";
15 answer = "";
16 }
17
18 /**
19 Sets the question text.
20 @param questionText the text of this question
21 */
22 public void setText(String questionText)
23 {
24 text = questionText;
25 }
26
27 /**
28 Sets the answer for this question.
29 @param correctResponse the answer
30 */
31 public void setAnswer(String correctResponse)
32 {
33 answer = correctResponse;
34 }
35
36 /**
37 Checks a given response for correctness.
38 @param response the response to check
39 @return true if the response was correct, false otherwise
40 */
41 public boolean checkAnswer(String response)
42 {
43 return response.equals(answer);
44 }
45
46 /**
47 Displays this question.
48 */
49 public void display()
50 {
51 System.out.println(text);
52 }
53 }
C. HW:
Should a class Quiz inherit from the class Question? Why or why not?
Now suppose you want to write a program that handles questions such as the following:
In which country was the inventor of Java born?
1. Australia
2. Canada
3. Denmark
4. United States
Which class will you create? How will you add the choices? Please create the class in repl.it or Codiva.io (Hint: see the diagram below)
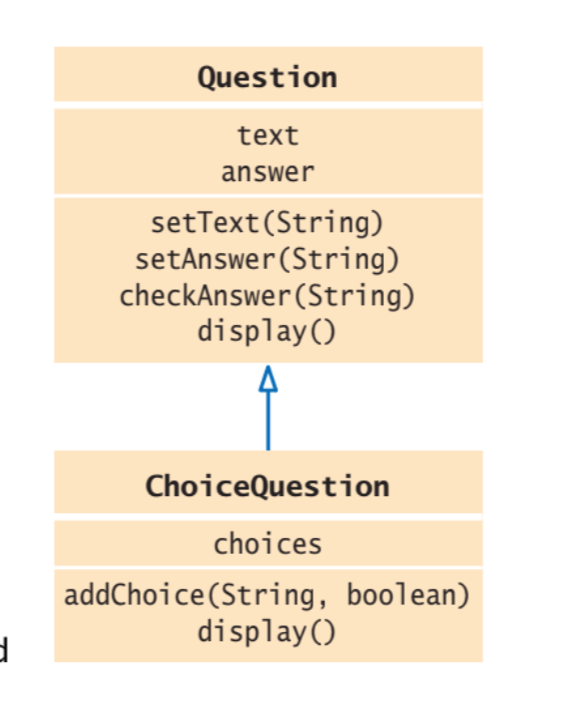