A. Introduction
After studying linear search and binary search, you can probably traverse an array easily. Before we look at the magic square, think about how you would get the sum of all elements in a 2-D array.
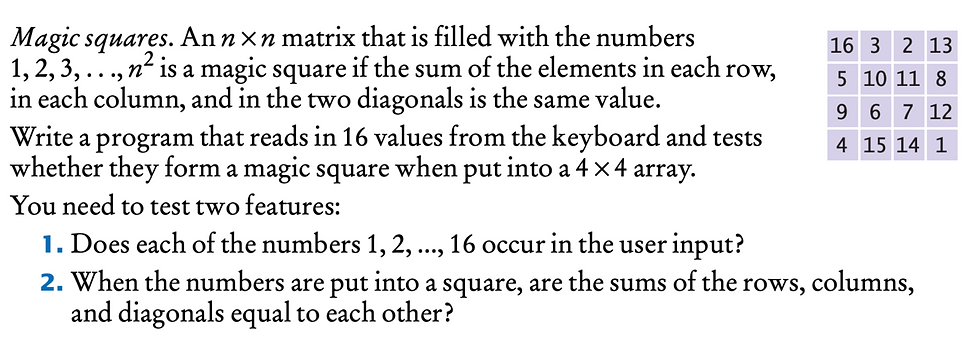
B. Discussion
How do we make sure 1, 2, ..., 16 are all in the square?
How to add up each row? each column? the two diagonals?
Is this similar to the game to Tic-Tac-Toe?
Swapping two elements in an arrays is useful. How can we swap two rows or two columns in a 2-D array? How to swap the top and bottom halves? How to swap the left and right halves?
For an n x n 2-D array, how to flip the array along the major or minor diagonal? Can you do that without creating a new array?
C. HW
Create the method below to return a Pascal Triangle. Call the method in main() to print out the Pascal Triangle when n=12.
Write code to rotate a 3 x 3 array 90 degrees clockwise.
Write code to rotate a 4 x 4 array 90 degrees counter clockwise.
In reality, any images are stored as a 2-D array. Can you create an algorithm to rotate an image 90 degrees clockwise? (You don't need the actual image, just think of it as an n x n array where n is in the range of hundreds or thousands.)